Introduction
Impersonation is a powerful feature that allows administrators or developers to act on behalf of users, often for troubleshooting or management purposes. When working with Supabase and Next.js, implementing impersonation can be straightforward, thanks to Supabase’s robust authentication system and Next.js’s serverless capabilities.
In this blog, we’ll explore how to set up impersonation in your Supabase project using Next.js. By the end, you’ll have a fully functional impersonation system to access and manage user-specific data seamlessly.
Why Impersonation?
Impersonation enables developers or admins to:
- Debug user-specific issues efficiently.
- Provide better support by seeing exactly what the user experiences.
- Access restricted data or perform actions on behalf of users.
With Supabase, this is achieved by generating custom JSON Web Tokens (JWTs) for temporary access.
Setting Up Impersonation in Supabase with Next.js
Step 1: Install Required Dependencies
To get started, install the required libraries:
npm install jsonwebtoken @supabase/supabase-js
jsonwebtoken
is used for generating custom JWTs.@supabase/supabase-js
is the official Supabase client for JavaScript.
Step 2: Configure Environment Variables
In your .env.local
file, add:
<code>NEXT_PUBLIC_SUPABASE_URL=<your-supabase-url>
SUPABASE_ANON_KEY=<your-anon-key>
SUPABASE_JWT_SECRET=<your-jwt-secret>
</code>
- The
SUPABASE_JWT_SECRET
is critical for generating custom JWTs.
Step 3: Create the API Route for Impersonation
Next.js’s API routes make it easy to create backend functionality. Here’s how to create an API route for impersonation:
import jwt from 'jsonwebtoken';
import { createServerDbClient } from '@/utils/supabaseClient';
export default async function handler(req, res) {
if (req.method === 'POST') {
const { userId } = req.body;
if (!userId) {
return res.status(400).json({ error: 'User ID is required.' });
}
try {
// Generate a custom JWT for impersonation
const payload = {
sub: userId,
role: 'authenticated',
exp: Math.floor(Date.now() / 1000) + 60 * 60, // 1-hour expiry
};
const token = jwt.sign(payload, process.env.SUPABASE_JWT_SECRET);
// Use the token to create a Supabase client
const supabase = createServerDbClient(token);
// Fetch user data via Supabase RPC or direct queries
const { data, error } = await supabase.rpc('get_user_email1', {
user_id: userId, // Replace with your actual RPC function and parameters
});
if (error) {
console.error('Error fetching user email:', error);
return res.status(500).json({ error: 'Failed to fetch user details.' });
}
// Return the impersonation token and user data
return res.status(200).json({ token, user: data });
} catch (error) {
console.error('Error impersonating user:', error);
return res.status(500).json({ error: 'Impersonation failed.' });
}
} else {
res.setHeader('Allow', ['POST']);
return res.status(405).end(`Method ${req.method} Not Allowed`);
}
}
Step 4: Secure the Impersonation API
- Authentication: Restrict this route to admin users or those with elevated privileges.
- Rate Limiting: Implement rate limiting to prevent abuse.
- Audit Logs: Log all impersonation requests for transparency and debugging.
Step 5: Client-Side Usage
Here’s how you can call the impersonation API from your client-side code:
async function impersonateUser(userId) {
const response = await fetch('/api/impersonate', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ userId }),
});
if (!response.ok) {
throw new Error('Failed to impersonate user');
}
const { token, user } = await response.json();
console.log('Impersonation Token:', token);
console.log('User Details:', user);
}
Key Takeaways
- JWT Expiry: Always set a short expiry time for impersonation tokens.
- Minimal Permissions: Limit the impersonation token’s scope to essential resources.
- Error Handling: Handle potential issues, such as invalid user IDs or token signing errors, gracefully.
Common Use Cases
- Admin Portals: Allow admins to troubleshoot user issues by impersonating accounts.
- Customer Support Tools: Equip support teams with the ability to view user-specific data.
- Debugging: Simplify debugging by replicating the user experience directly.
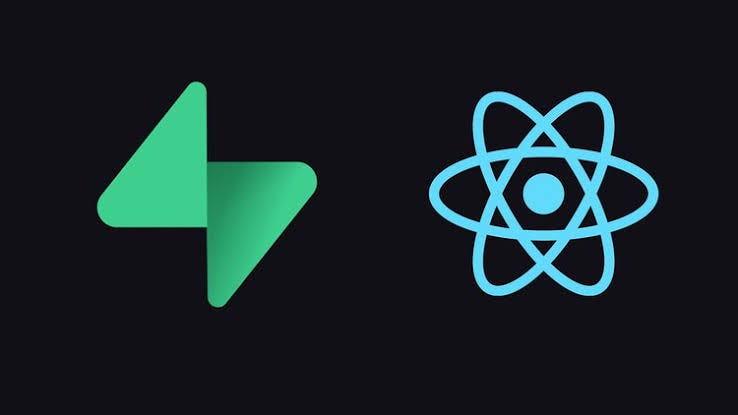
Conclusion
Impersonation is a game-changer for improving user support and debugging. By combining Supabase’s authentication capabilities with Next.js’s flexibility, you can implement a secure and efficient impersonation system in your applications.
Start integrating impersonation into your Supabase-powered projects today, and let us know how it helps streamline your development and support processes!